Build Adaptive Card Extension with SPFx
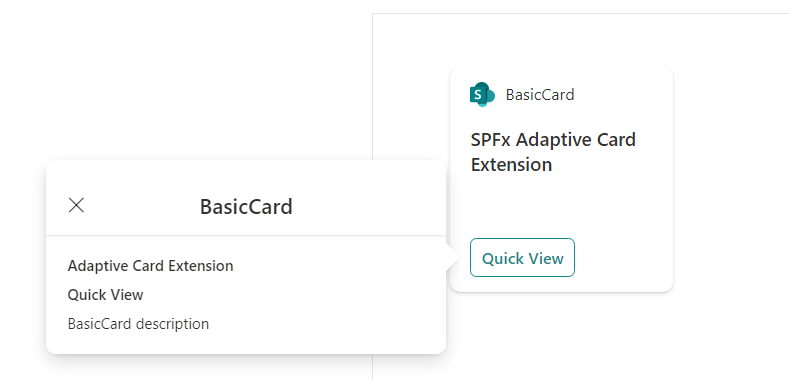
Overview
SPFx v1.13 supports Adaptive Card Extensions (ACEs) component type which enables developers to build a rich experience for Viva Connections’ Dashboards and SharePoint Pages.
In this article, we will explore the ACE component types and build our first SPFx experience with ACE.
Adaptive Card Extensions Overview
Adaptive Card Extensions (ACE) uses Adaptive Card Framework with declarative JSON schema to generate UI, so the developer can focus on the business logic rather than thinking of complex JSON building on their own.
ACEs support below list of templates:
- Basic Card Template
- Image Card Template
- Primary Text Template
Install the latest beta release by running the below command:
npm install @microsoft/generator-sharepoint@next --global
Scaffold Adaptive Card Extensions (ACE) solution
Start the scaffolding process by running the below command:
yo @microsoft/sharepoint
Test Basic Card Template
Follow the below steps to test the basic card template:
-
Execute the below command to run the solution:
gulp serve -l --nobrowser
-
On the browser, navigate to SharePoint hosted workbench.
-
Add ACE to the page.
-
Click on Quick View to see the basic card popping out.
-
Click the Edit icon to see the property pane.
Note: ACE interactions are only supported when the Workbench or Page is in Preview or Read mode.
Code Walkthrough
On the command prompt, type code .
to open the solution in the code editor (e.g. Visual Studio Code).
ACE Class
The file src\adaptiveCardExtensions\basicCard\BasicCardAdaptiveCardExtension.ts
contains the definition of an ACE, which extends from the BaseAdaptiveCardExtension
class.
export default class BasicCardAdaptiveCardExtension extends BaseAdaptiveCardExtension<
IBasicCardAdaptiveCardExtensionProps,
IBasicCardAdaptiveCardExtensionState
> {
// ...
}
Here we can optionally implement two generics:
- TProperties: Set of persisted properties of the component (a property bag)
- TState: State of ACE
Render ACE
The virtual renderCard()
method returns the string identifier to the registered view. This method is invoked during the initial render of the Card view.
protected renderCard(): string | undefined {
return CARD_VIEW_REGISTRY_ID;
}
If this method is commented, quick view will not render. Unlike with the Card view, there is no default Quick view.
Register View for ACE
View must be registered within class’ constructor or onInit()
method before we use it.
public onInit(): Promise<void> {
...
this.cardNavigator.register(CARD\_VIEW\_REGISTRY\_ID, () => new CardView());
this.quickViewNavigator.register(QUICK\_VIEW\_REGISTRY\_ID, () => new QuickView());
return Promise.resolve();
}
Card View
Card view can be found at src\adaptiveCardExtensions\basicCard\cardView\CardView.ts
It must extend from any of below base classes:
- BaseBasicCardView
- BaseImageCardView
- BasePrimaryTextCardView
Two generics for the properties and state objects are shared between the view and the ACE.
data
The data
getter is the only method that must be implemented by a Card view.
public get data(): IBasicCardParameters {
return {
primaryText: strings.PrimaryText
};
}
Note: The return type is unique to the parent class of the View.
cardButtons
The cardButtons
property determines the number of buttons that appear on the Card and actions to perform when clicked.
public get cardButtons(): [ICardButton] | [ICardButton, ICardButton] | undefined {
return [
{
title: strings.QuickViewButton,
action: {
type: 'QuickView',
parameters: {
view: QUICK_VIEW_REGISTRY_ID
}
}
},
{
title: 'Microsoft',
action: {
type: 'ExternalLink',
parameters: {
target: 'https://www.microsoft.com'
}
}
}
];
}
onCardSelection This method determines what will happen when the Card is clicked.
public get onCardSelection(): IQuickViewCardAction | IExternalLinkCardAction | undefined {
return {
type: 'ExternalLink',
parameters: {
target: 'https://www.bing.com'
}
};
}
Quick View
Quick view can be found at src\adaptiveCardExtensions\basicCard\quickView\QuickView.ts
.
Quick views extend the BaseAdaptiveCardView
base class with below three optional generics:
- TProperties: Interface used by persisted properties of the ACE (a property bag).
- TState: Set of stateful data the View needs to render.
- TData: The type returned from the data() getter method.
template()
The template()
getter returns valid Adaptive Card template JSON.
public get template(): ISPFxAdaptiveCard {
return require('./template/QuickViewTemplate.json');
}
The properties on the object returned from the data getter will automatically be mapped to the bound template slot.
public get data(): IQuickViewData {
return {
subTitle: strings.SubTitle,
title: strings.Title,
description: this.properties.description
};
}
The template slot can be found at src\adaptiveCardExtensions\basicCard\quickView\template\QuickViewTemplate.json
.
{
"type": "TextBlock",
"text": "${description}",
"wrap": true
}
Summary
SPFx v1.13 supports Adaptive Card Extensions (ACEs) component type which enables developers to build a rich experience for Viva Connections’ Dashboards and SharePoint Pages.
Leave a comment