Action Handlers in Adaptive Card Extension with SPFx
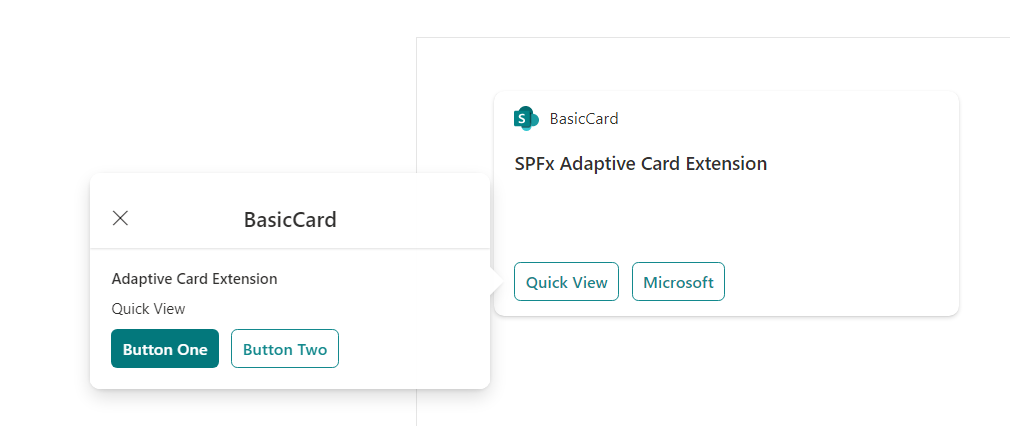
Overview
In the previous article Build Adaptive Card Extension with SPFx we explored the ACE component types and build our first SPFx experience with ACE.
In this article, we will extend it further and write our action handlers.
Add Actions
We will start by adding actions to Quick View template at src\adaptiveCardExtensions\basicCard\quickView\template\QuickViewTemplate.json
{
"schema": "http://adaptivecards.io/schemas/adaptive-card.json",
"type": "AdaptiveCard",
"version": "1.2",
"body": [
{
"type": "TextBlock",
"weight": "Bolder",
"text": "${title}"
},
{
"type": "ColumnSet",
"columns": [
{
"type": "Column",
"items": [
{
"type": "TextBlock",
"weight": "Bolder",
"text": "${subTitle}",
"wrap": true
}
]
}
]
},
{
"type": "TextBlock",
"text": "${description}",
"wrap": true
}
]
}
We now have 2 buttons added to our Quick View. However, they do not respond to a button click.
Action handlers
Actions are handled by the views where they’re defined. The button click initiates the Action.Submit action when clicked which can be handled inside onAction()
method.
Open QuickView.ts
file at src\adaptiveCardExtensions\basicCard\quickView\QuickView.ts
to handle button click events.
import { ISPFxAdaptiveCard, BaseAdaptiveCardView } from '@microsoft/sp-adaptive-card-extension-base';
public onAction(action: IActionArguments): void {
if (action.type === 'Submit') {
const { id, message } = action.data;
switch (id) {
case 'button1':
case 'button2':
this.setState({
description: message
});
break;
}
}
}
Update data
getter method to set the description from the state.
public get data(): IQuickViewData {
return {
subTitle: strings.SubTitle,
title: strings.Title,
description: this.state.description
};
}
Summary
Actions are handled by the views where they are defined. The button click initiates the Action.Submit
action when clicked which can be handled inside onAction()
method.
Leave a comment