Using Azure OpenAI APIs in Bots with Teams Toolkit
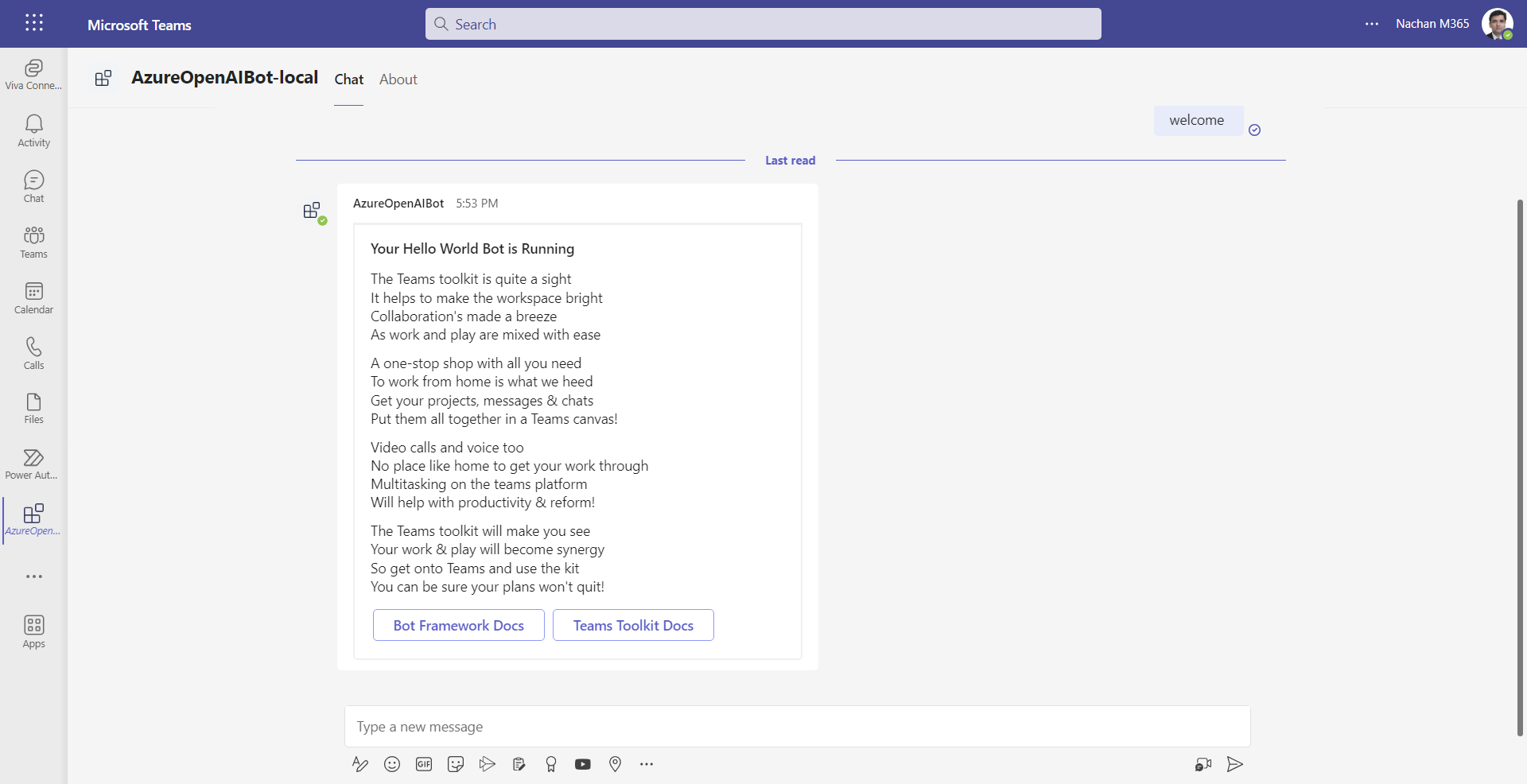
Overview
Teams Toolkit helps developers develop apps for Microsoft Teams. Adding AI capabilities to your Teams app can be easily done with Azure OpenAI.
In this article, we will explore adding Azure OpenAI API capabilities to bots created with Teams Toolkit with easy-to-follow and step-by-step instructions.
Teams Toolkit Overview
Teams Toolkit is available as an extension for Visual Studio Code and Visual Studio 2022 to help developers create, debug, and deploy Microsoft Teams apps.
In Visual Studio Code, it can be installed as an extension. Follow the below steps to install:
- Open Visual Studio Code.
- Click View > Extensions.
-
Find and install Teams Toolkit.
Create a Bot solution
Follow the steps below to create a bot solution with Teams Toolkit.
- Open Visual Studio Code.
- From the left menu, click Teams Toolkit.
- Choose to Create a new app.
-
Select an option: Create a new Teams app.
-
Select Command bot to create.
- Choose a programming language as TypeScript.
- Select a folder location to scaffold the project.
- Enter the Application Name as OpenAIChatBot.
Once the project is scaffolded, you can select Run and Debug from the left menu. Choose the run profile and test the bot solution.
Azure OpenAI npm package
We will use the Azure OpenAI npm package available at https://www.npmjs.com/package/@azure/openai in this solution. The OpenAI Node.js library provides convenient access to the OpenAI API from Node.js applications.
Install the package by running the below command:
npm install @azure/openai
Azure OpenAI
Follow the below steps to set up Azure OpenAI:
- Create an Azure OpenAI service in the Azure portal.
- In the Azure AI Studio, From the left navigation under Management , click Models.
- Search and select the text-davinci-003 model.
- Click deploy.
The model will now be available under the Deployments section. Select the text-davinci-003 model and click Open in Playground.
In the Completions playground, click View code and note down the Endpoint and Key.
We will store the Endpoint and Key in our solution as a configuration in the bot\src\internal\config.ts file.
const config = {
botId: process.env.BOT_ID,
botPassword: process.env.BOT_PASSWORD,
endpoint: 'https://XXXX-openai.openai.azure.com/',
azureApiKey: 'XXXX'
};
export default config;
Command Handler implementation in Bot
Start by adding below import to teamsBot.ts
.
import { OpenAIClient, AzureKeyCredential } from "@azure/openai";
Implement the welcome
command handler as follows:
...
case "welcome": {
// Call Azure OpenAI to get the assessment questions
const prompt = [`Generate a poem on Microsoft Teams toolkit`];
// You will need to set these environment variables or edit the following values
const endpoint = config.endpoint;
const azureApiKey = config.azureApiKey;
const client = new OpenAIClient(endpoint, new AzureKeyCredential(azureApiKey));
const deploymentId = "text-davinci-003";
const result = await client.getCompletions(deploymentId, prompt, { maxTokens: 4000 });
var choiceText = "";
for (const choice of result.choices) {
choiceText = choice.text;
}
this.openAIOutputObj.openAIOutput = choiceText;
// Fix missing quotation marks on keys in JSON
choiceText = choiceText.replace(/(['"])?([a-zA-Z0-9_]+)(['"])?:([^\/])/g, '"$2":$4');
const card = AdaptiveCards.declare<OpenAIDataInterface>(rawWelcomeCard).render(this.openAIOutputObj);
await context.sendActivity({ attachments: [CardFactory.adaptiveCard(card)] });
break;
}
...
Test the bot
From the Visual Studio Code, select Run and Debug from the left menu. Choose the run profile and test the bot solution.
Summary
Adding AI capabilities to your Teams app can be easily done with Azure OpenAI. This can be easily extended to bots with Teams Toolkit to provide engaging experience for the end-users.
Code Download
The code developed during this article can be found here.
Leave a comment